前端之虎陈随易
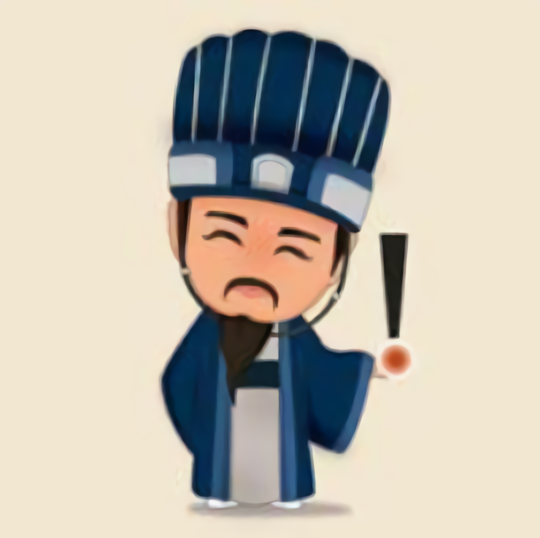
electron-vite is a build tool that aims to provide a faster and leaner development experience for Electron. It consists of five major parts:
A build command that bundles your code with Vite, and able to handle Electron's unique environment including Node.js and browser environments.
Centrally configure the main process, renderers and preload scripts Vite configuration, and preconfigure for Electron's unique environment.
Use fast Hot Module Replacement(HMR) for renderers, and the main process and preload scripts support hot reloading, extremely improving development efficiency.
Optimize asset handling for Electron main process.
Use V8 bytecode to protect source code.
electron-vite is fast, simple and powerful, designed to work out-of-the-box.
You can learn more about the rationale behind the project in the Introduction section.
Pre-requisites
Requires Node.js version 18+, 20+ and Vite version 4.0+
npm i electron-vite -D
npm i electron-vite -D
In a project where electron-vite is installed, you can use electron-vite
binary directly with npx electron-vite
or add the npm scripts to your package.json
file like this:
{
"scripts": {
"start": "electron-vite preview", // start electron app to preview production build
"dev": "electron-vite dev", // start dev server and electron app
"prebuild": "electron-vite build" // build for production
}
}
{
"scripts": {
"start": "electron-vite preview", // start electron app to preview production build
"dev": "electron-vite dev", // start dev server and electron app
"prebuild": "electron-vite build" // build for production
}
}
You can specify additional CLI options like --outDir
. For a full list of CLI options, run npx electron-vite -h
in your project.
Learn more about Command Line Interface.
When running electron-vite
from the command line, electron-vite will automatically try to resolve a config file named electron.vite.config.js
inside project root. The most basic config file looks like this:
// electron.vite.config.js
export default {
main: {
// vite config options
},
preload: {
// vite config options
},
renderer: {
// vite config options
}
}
// electron.vite.config.js
export default {
main: {
// vite config options
},
preload: {
// vite config options
},
renderer: {
// vite config options
}
}
Learn more about Config Reference.
When using electron-vite to bundle your code, the entry point of the Electron application should be changed to the main process entry file in the output directory. The default outDir
is out
. Your package.json
file should look something like this:
{
"name": "electron-app",
"version": "1.0.0",
"main": "./out/main/index.js"
}
{
"name": "electron-app",
"version": "1.0.0",
"main": "./out/main/index.js"
}
Electron's working directory will be the output directory, not your source code directory. So you can exclude the source code when packaging Electron application.
Learn more about Build for production.
Run the following command in your command line:
npm create @quick-start/electron@latest
npm create @quick-start/electron@latest
yarn create @quick-start/electron
yarn create @quick-start/electron
pnpm create @quick-start/electron
pnpm create @quick-start/electron
Then follow the prompts!
✔ Project name: … <electron-app>
✔ Select a framework: › vue
✔ Add TypeScript? … No / Yes
✔ Add Electron updater plugin? … No / Yes
✔ Enable Electron download mirror proxy? … No / Yes
Scaffolding project in ./<electron-app>...
Done.
✔ Project name: … <electron-app>
✔ Select a framework: › vue
✔ Add TypeScript? … No / Yes
✔ Add Electron updater plugin? … No / Yes
✔ Enable Electron download mirror proxy? … No / Yes
Scaffolding project in ./<electron-app>...
Done.
You can also directly specify the project name and the template you want to use via additional command line options. For example, to scaffold an Electron + Vue project, run:
# npm 6.x
npm create @quick-start/electron my-app --template vue
# npm 7+, extra double-dash is needed:
npm create @quick-start/electron my-app -- --template vue
# yarn
yarn create @quick-start/electron my-app --template vue
# pnpm
pnpm create @quick-start/electron my-app --template vue
# npm 6.x
npm create @quick-start/electron my-app --template vue
# npm 7+, extra double-dash is needed:
npm create @quick-start/electron my-app -- --template vue
# yarn
yarn create @quick-start/electron my-app --template vue
# pnpm
pnpm create @quick-start/electron my-app --template vue
Currently supported template presets include:
JavaScript | TypeScript |
---|---|
vanilla | vanilla-ts |
vue | vue-ts |
react | react-ts |
svelte | svelte-ts |
solid | solid-ts |
See create-electron for more details.
create-electron is a tool to quickly start a project from a basic template for popular frameworks. Also you can use a tool like degit to scaffold your project with the template electron-vite-boilerplate.
npx degit alex8088/electron-vite-boilerplate electron-app
cd electron-app
npm install
npm run dev
npx degit alex8088/electron-vite-boilerplate electron-app
cd electron-app
npm install
npm run dev
If you suspect you're running into a bug with the electron-vite
, please check the GitHub issue tracker to see if any existing issues match your problem. If not, feel free to fill the bug report template and submit a new issue.